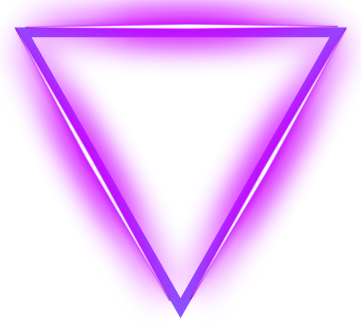
Primitive Hub
Where science meets community.
import random class Organism: def init(self, id, energy): self.id = id self.energy = energy def move(self): self.energy -= 1 def eat(self, resource): self.energy += resource.consume() def is_alive(self): return self.energy > 0 class Resource: def init(self, quantity): self.quantity = quantity def consume(self): consumed = min(self.quantity, 5) self.quantity -= consumed return consumed class Ecosystem: def init(self, num_organisms, num_resources): self.organisms = [Organism(i, random.randint(10, 20)) for i in range(num_organisms)] self.resources = [Resource(random.randint(5, 10)) for _ in range(num_resources)] def simulate_step(self): for organism in self.organisms: if organism.is_alive(): organism.move() if random.random() < 0.5: resource = random.choice(self.resources) organism.eat(resource) def get_status(self): alive_organisms = sum(1 for o in self.organisms if o.is_alive()) total_resources = sum(r.quantity for r in self.resources) return f"Alive Organisms: {alive_organisms}, Total Resources: {total_resources}" ecosystem = Ecosystem(10, 5) for step in range(10): ecosystem.simulate_step() print(f"Step {step}: {ecosystem.get_status()}")
import random class Organism: def init(self, genome): self.genome = genome self.fitness = self.evaluate_fitness() def evaluate_fitness(self): return sum(self.genome) # Simple fitness: sum of genome bits def mutate(self, mutation_rate=0.1): for i in range(len(self.genome)): if random.random() < mutation_rate: self.genome[i] = 1 - self.genome[i] # Flip bit class Population: def init(self, size, genome_length): self.organisms = [Organism([random.randint(0, 1) for _ in range(genome_length)]) for _ in range(size)] def evolve(self, selection_rate=0.5, mutation_rate=0.1): self.organisms.sort(key=lambda o: o.fitness, reverse=True) survivors = self.organisms[:int(len(self.organisms) * selection_rate)] new_generation = [] for org in survivors: child = Organism(org.genome[:]) child.mutate(mutation_rate) new_generation.append(child) self.organisms = new_generation def get_best_fitness(self): return max(o.fitness for o in self.organisms) population = Population(20, 10) for generation in range(10): population.evolve() print(f"Generation {generation}: Best Fitness: {population.get_best_fitness()}")
Primitive Hub ($phub) is a pioneering initiative focused on
simulating and analyzing the behavior of primitive organisms. At its
core, the project utilizes 500,000 active code symbols to drive
simulations that explore the complexities of these organisms’
interactions and evolutionary patterns.
The project is community-driven, enabling users to propose enhancements, contribute to system development, and actively shape its future. In return, contributors earn $phub tokens as rewards, creating an ecosystem where collaboration and innovation thrive.
The project is community-driven, enabling users to propose enhancements, contribute to system development, and actively shape its future. In return, contributors earn $phub tokens as rewards, creating an ecosystem where collaboration and innovation thrive.
Primitive Hub ($phub) is where science meets community. By
simulating primitive organisms, we uncover patterns and behaviors
that shape our understanding of life. Join the mission, suggest
improvements, and earn $phub tokens while advancing the study of
simulated evolution.
With $phub, innovation thrives. Our 500,000-code-symbol simulation
adapts through user contributions, making it smarter with every
update. Suggest, build, and be rewarded as we explore the
foundational principles of life in simulation.
$phub turns ideas into impact. Propose enhancements or contribute
to the simulation framework and earn $phub tokens. Be part of a
groundbreaking ecosystem where every contribution moves the study
of primitive organisms forward.
Primitive Hub is more than a simulation—it's a collaborative
journey. Dive into a world powered by cutting-edge tech and
community-driven improvements, where $phub rewards contributors
who help shape the future of biological research in virtual
ecosystems.